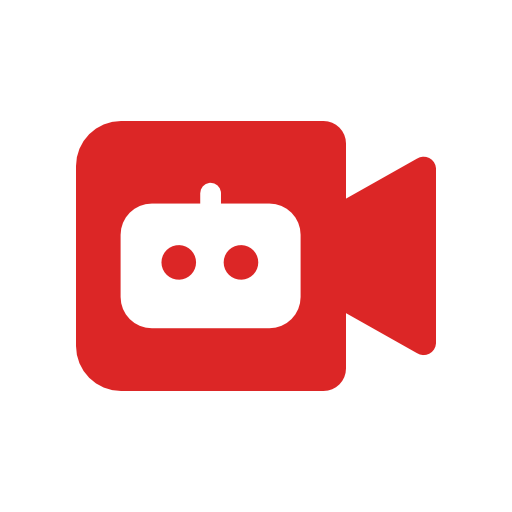
Generate a Video
/script-to-video
There are two primary methods for interacting with the /script-to-video
endpoint: polling and webhooks. This guide will walk you through how to send a request and receive a response using both approaches. By the end of this guide, you will be able to choose the appropriate method and handle responses effectively for your use case.
Polling
The easiest way to check the status of your request is by polling based on the apiFileId
. You can include this ID as a query parameter in the /get-file
endpoint, which will return the current status of the request, how long it has been running, and other relevant details. Keep in mind that the video export time will typically be around the same length as the video itself. Below is an example of how to implement polling logic. This method allows you to continually monitor the progress of your request without needing to rely on webhooks.
INITIALIZE validResponse TO false
SET startTime TO current time
WHILE (current time - startTime) < timeout DO
TRY
MAKE GET request TO "/get-file" WITH headers
Authorization: "Bearer apiKey"
Content-Type: "application/json"
EXTRACT responseBody FROM response
IF responseBody.loadingState IS "FULFILLED" THEN
SET validResponse TO true
BREAK the loop
END IF
CATCH error
THROW "Error while polling"
END CATCH
WAIT intervalTime BEFORE NEXT POLL
END WHILE
Webhooks
In addition to polling, you can also use webhooks to receive a notification when your video request is complete. With webhooks, you simply provide a callback URL when making the initial request to the /script-to-video
endpoint. Once the video is generated, the API will send a POST
request to your webhook URL, containing a JSON body with the apiFileId
and apiFileSignedUrl
. This approach eliminates the need to continuously check for updates, making it a more efficient option for longer video exports or when you don’t need immediate feedback. By using webhooks, you can handle the completion of video processing automatically and perform actions as soon as the video is ready.
{
"script": "Machine learning is a technique where algorithms learn from data to make predictions or decisions without explicit programming... It powers applications like image recognition, natural language processing, and predictive analytics, continuously improving as it processes more data.",
"webhookUrl": "https://your-webhook-url.com/endpoint"
}